by Michael Cropper | Jan 24, 2020 | Developer, SEO |
Ok. Back to basics. It’s rare these days that any developer working in the real world has to write a basic HelloWorld.java, compile the file with the core Java Compiler (javac, the Java Programming Language Compiler) and run the file using the command line ‘java’ command. But hey, sometimes things are just fiddly to debug and you need to get back to the real basics and say HelloWorld.
Honestly, I’m mainly writing this blog post for myself as I can never remember how to do this as it is something that I haven’t had to do in many years, because you know, we have things called IDEs, Web Applications, Automated Deployments and just modern technology in general which is the space I tend to work in.
Just a general note, the common reason why you’ll need to do things like this is because your Local à Dev à Test à Live Environments are slightly different. Different enough to cause issues that you’ll only notice while you migrate your applications through the development lifecycle.
How to Create a HelloWorld.java File with a Main Method
For the purpose of this blog post, let’s forget we’re in the 20th Century using a modern IDE, let’s get Notepad open.
For the purpose of this blog post, I’m going to use a real world example that I was debugging, specifically when trying to figure out why the ‘reasonably recent’ Java 8 functionality on one application wasn’t working on one application server, which had recently had Java 8 installed with Tomcat 7 which was installed via WHM > cPanel’s Easy Apache 3. The LocalDate.now() function is only available from Java 8, and a specific release of Java 8, just to add to the confusion. None of which is really relevant for this blog post, but it puts into context why you sometimes need to go back to real basics and to try and figure out where the problem ultimately lies.
Sometimes you just need to strip back the many layers of applications, get back to the core and build up from there to identify where the problem is. Anyhow, back to where we were.
Basically create this file and save the filename as HelloWorldMain.java;
package PlayGround;
import java.time.LocalDate;
 
public class HelloWorldMain {
public static void main(String[] args) {
System.out.println("LocalDate.now(): " + LocalDate.now());
}
}
Yes that’s right, pure, core, Java. No frameworks. No libraries. No helpers. Nothing. Welcome to the 80s.
The reason why we’re creating a Main method is to ensure that when we run the compiled code, as detailed later on, it will automatically run the Main method and print the information you require to the command line console.
How to Compile Your HelloWorld.java File to Create a HelloWorld.class File
Ok, now you’ve created your HelloWorldMain.java file as outlined in the previous step, it’s time to compile this into executable code. As you’ll know, you can’t run .java files as they are (unlikely say .html files), instead you need to compile your .java files into .class files using the Java Development Kit (aka. JDK) that is installed on your machine (local machine or server).
To compile your .java file, firstly you need to open the Command Prompt (on Windows) or SSH into your Linux server. Using your command line skills (simple right…?), navigate to the location of your file and compile your .java file by running the following command;
javac HelloWorldMain.java
Awesome, you should now have a HelloWorldMain.class file created in the same directory you are currently in.
But… things aren’t always quite as they seem. We’ve not gone into any of the details yet about where you’ve just run the Java compiler command. Windows? Linux? Now this is where things get interesting. Let’s say you’re developing on a Windows machine, and you’re deploying on a Linux server. Immediately you’ve got a discrepancy.
What version of Java are you running on your Windows machine?
What version of Java are you running on your Linux machine?
What other crazy-ass things have you got configured that may be getting in the way in either environment? Hopefully nothing, but keep that in mind. Often things are configured in the background that you may not be aware of, particularly whenever you’ve used handy installers and other software to manage the installation of your environments.
So, let’s get back to the basics and find out the environments we’re working in.
Check the Java Version
On the machines you’re working on, check what version of Java is currently the priority by running the command;
java -version
You can also view on Linux the Environment Variables which can help to identify what is setup by running the command;
printenv
(aka. the Print Environment Variables command)
And on Windows you can view your Environment Variables by opening the Command Prompt ( aka. Windows Start Menu > Run > Then type ‘cmd’ without the quotes and press go ) or ( Just press Windows Key + R ), then type;
set
Which will output your Environment Variables.
Check the Java Alternatives
On Linux machines you can configure multiple versions of Java to run via the ‘alternatives’ feature. This essentially allows you to install multiple Java versions alongside each other without having to worry about the specifics. This allows you to have multiple versions of Java installed, and still configure a default.
Which basically means that when you run any default java command that it will run against whatever alternative has been configured to point at the different versions of Java installed on your Linux box.
To check this, simply run the command;
sudo alternatives --config java
Which will allow you to configure which is the default Java version – according to the Linux environment.
This is an important point to note, since an application (for example Tomcat…) can actually still override this locally within the application configuration files and hence completely ignore what you set at the base server level. The joys and flexibility (aka. frustrations…) of dealing with Linux.
How to Run Your HelloWorld.class File From the Command Line
Ok, so now we’ve covered a fair amount of the ‘basics’, let’s take a look at actually running the file we’ve just created.
Basically just run this command (with tweaks) against the file that is hosted against your own Linux server;
java -cp /home/{Root Directory for User }/public_html/WEB-INF/classes/ PlayGround.HelloWorldMain
by Michael Cropper | Feb 15, 2019 | SEO |
When it comes to technology, technology should work for you, you should not be a slave to your technology. And oh-my-god, Windows have got things SOOOOOO wrong with their automatic update system in Windows 10. For the average home user, you’ll probably be thankful that things are simply taken care of for you, and that’s great. Security is hugely important and this is where the Windows 10 automatic updates come in very handy. But Microsoft seem to have forgot one key thing with these updates and that is that not everyone is an average user and Windows is used by businesses that require stability and control.
The amount of times I have gone to make a brew (that’s a ‘Cup of Tea’ for the Southerners reading…), or turned by back for only a few minutes to come back to my machine and find that bloody Windows 10 has turned itself off, in the middle of the flipping day, in the middle of when I was doing something!!! Thankfully, I have a good habit of saving every 2 seconds so I’ve never lost anything, touch wood, but by god is this annoying.
What’s more annoying is the fact that when Windows 10 built the feature as part of this update to helpfully re-open all the things you had open, they forgot one thing…. not everything you use is a bloody Microsoft product! The amount of things that people use in the working day, particularly myself with the amount of techie things I have open at any one point, that simple turning things off and back on again causes more problems than it solves (ironic for an IT product!). Even on powerful machines, for users who have things on the go, it’s going to take you a good 10, 15, 30 minutes to get everything back up and running that you were working on. It’s such a time wasting activity that I’ve decided to outline the steps required to just turn everything off for Windows 10 automatic security updates…
Just do this;
Open the Run Dialog
Press Windows Key + R – This will open the Windows Run window where you can type handy shortcuts to run programs
Open Windows Services
Type services.msc into the available field then click OK. This will open the Windows Services settings where you can control a lot of the background processes and tasks that run on your system.
Find ‘Windows Update’
In the rather large list of services, find the ‘Name’ of ‘Windows Update’ and Select It. Then Right Click and select Properties
Stop the Service if it is Running and Disable it on Startup
Simple, job done. No more annoying, interrupting, ill-thought-out Windows 10 automatic updates at inconvenient times!
Word of Warning
Don’t do any of the above if you aren’t sure what you are doing. Changing things within your Services can really mess up things that you would want to work.
Clearly security and feature updates are important and you need to schedule time to do this. So don’t simply turn this off and never run security updates again, that would be bad. Schedule some time in, on a regular basis, at your convenience to run the security updates. Simple start the service and the rest will take care of itself.
Hope the above is some use to people going through the same pain…
by Michael Cropper | Apr 13, 2017 | Client Friendly, Sector - Travel, Hostpitality and OTAs, SEO |
Travel is a competitive market, so it is important that your brand stands out from your competitors when customers are searching on Google for queries such as “Holidays to Kefalonia” and similar review type queries. Rich snippets are a great way to highlight your brand amongst your competitors which can generate additional traffic to your website from Google, leading to increased sales and enquiries.
So what are Review Rich Snippets and when do they show?
Rich Snippets are a form of structured data that simply helps Google to understand what the content on your website is about. There are Rich Snippets for a variety of areas and the one we are going to cover today is specific to highlighting review information on Google. What this means in practice is that when customers are searching for queries such as “Holidays to Kefalonia” or “Holidays to Kefalonia Reviews” there is a greater chance that Google will highlight your website with stars next to your listing as can be seen in the image below;
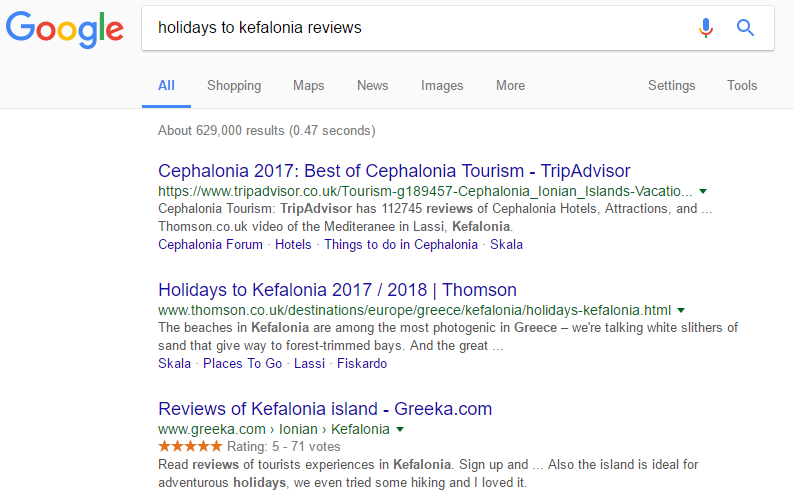
As you can see here, your eyes are instantly drawn to the listing that stands out from the others with the star ratings. In essence Review Rich Snippets require each individual Review to be marked up along with aggregating this data into an overall review based on all the review data. This information can be from your previous customers who have been on one of your holiday packages, so it is essential to be collecting this data from your customers at the earliest available opportunity.
How to Mark Up Review Rich Snippets
There are many ways to marl up Review Rich Snippet content which gets rather technical, so we’re only going to skim over this information as we don’t want to bamboozle you with the finer technical details. For the purposes of this blog post we’ll use the Greeka.com website as an example and take a look at what they have done to implement Review Rich Snippets on their travel website.
Firstly what you’ll notice when you visit their website is a listing of review information as you would expect to see as a user;
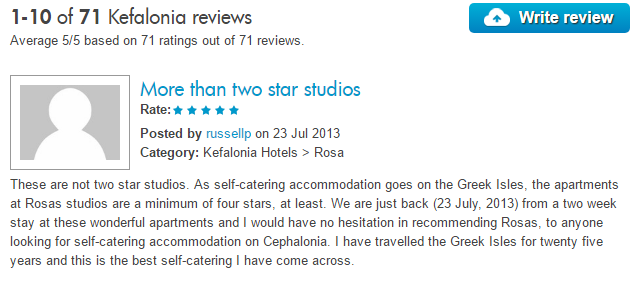
What’s important to note here is two pieces of information. Firstly, you will see the overall reviews listing at the very top which states that there are 71 reviews in total with the average being 5/5 for the reviews that have been left. Then beneath that you’ll notice an individual review that has been left by a customer. As a user, you’ll never notice Review Rich Snippets are there which is exactly why they exist. Review Rich Snippets for travel websites are in the code in the background which is telling Google that there are reviews associated with this specific item such as a holiday package, a resort, a restaurant or a specific hotel for example.
Here’s what this looks like in the background, the code below relates to the aggregate reviews which are what is showing on Google;

What you’ll notice is that there has been specific pieces of code wrapped around the content on the page which state that the destination has an average review of 5/5 from 71 people. When you read through the code you’ll notice there are specific pieces of information such as ‘best’ and ‘average’ and ‘votes’ etc. It is this information and more that Google is using to generate the visible star ratings on the Google search results that customers see when they are searching for travel companies.
Likewise you’ll also notice when reading through the code on the page that each individual review also has specific Review Rich Snippet data marked up around it too as you can see in the image below;
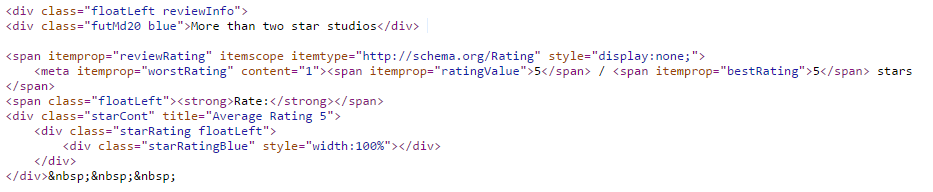
With information such as ‘reviewRating’ and ‘worstRating’ and ‘ratingValue’ for example being some of the key pieces of information Google requires to be able to see how the overall review ratings have been calculated.
Ok, that’s enough code for now. In essence, to get Review Rich Snippets and star ratings showing on Google it is important that you implement the code correctly which ties in closely with the technology that is powering your website. There is no one-size-fits-all approach here as every website is different.
If you’d like to implement Review Rich Snippets on your travel company website then get in touch and we’ll work closely with you to implement Review Rich Snippets so you can stand out from your competitors and boost website traffic and sales.
by Michael Cropper | Feb 14, 2017 | Client Friendly, Security, SEO, WordPress |
You may have recently received an email from Google Search Console warning you that your website is being flagged as Non-Secure Collection of Passwords as can be seen below;
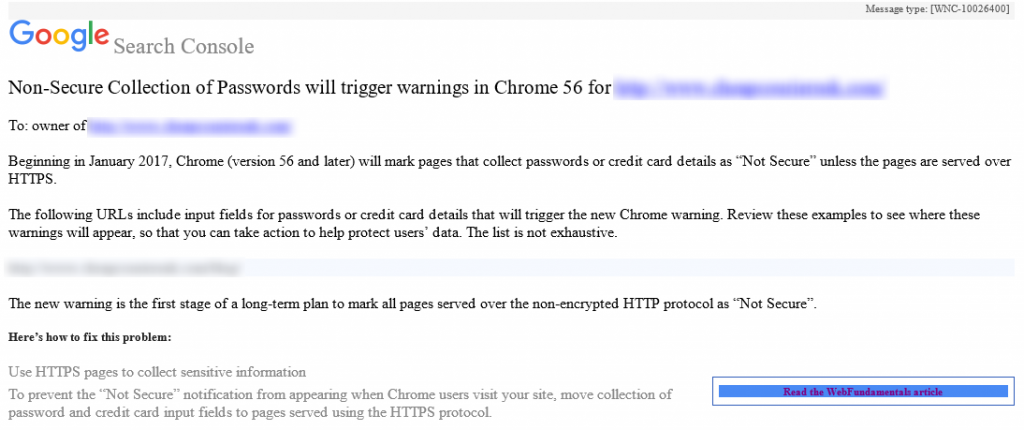
If you have been sent a message like this, you need to act before it is too late. You have received this message because your website is running over HTTP instead of HTTPS on pages that you collect sensitive information. Whenever either you or your users enter sensitive information on any website using HTTP, i.e. http://www.contradodigital.com/wp-login.php then this information can be seen in transit by anyone listening in on the network.
What you need to do
The solution to resolving these warnings is actually relatively simple. If you want to have a go at this yourself, then make sure you claim your free SSL certificate and update your website accordingly. If you need any help implementing this then get in touch and we can help you with the process.
by Michael Cropper | Jan 12, 2016 | Digital Strategy, Future, News, SEO |
Welcome to 2016. After reviewing the physical post received for the first week back in the office, I can only sit here with my head in my hands pondering why businesses are still failing to grasp how fast the world is really moving around them and adapt accordingly. We’ve our usual tips and advice blog post on their way for what you need to be focusing on in 2016 for your digital strategy, but in the meantime I feel the need to express my opinion on the state of what can only be described as utter junk that had been posted out to me and the company. Please, just stop.
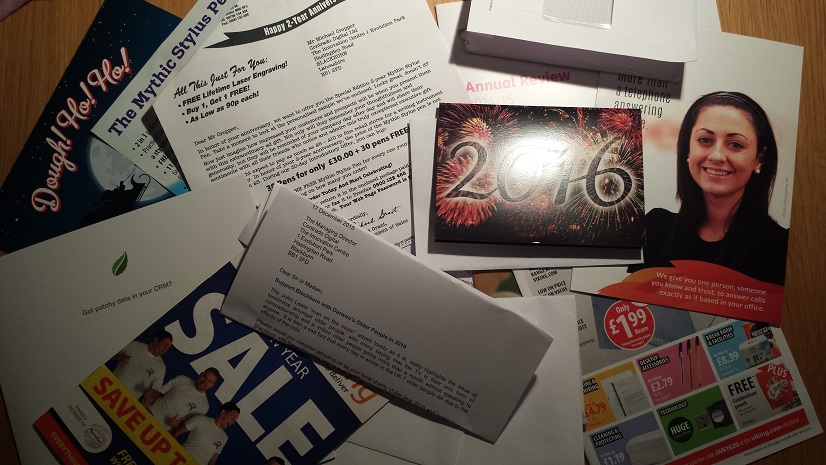
As you can see above, this is just a small selection of the garbage that was received, promotional pens labelled as “The Mythic Stylus Pen”, OK, I’m sure I know what one of these is, it’s not a unicorn walking down the street of Manchester blowing rainbows from its backside for f. sake. Sale catalogues, promotional material, wall planners, seriously who uses wall planners in 2016? Come on folk, please stop this. All of this junk received went straight into the bin. Stop wasting your money on junk and start to market your business effectively through digital channels. We live in a digital world, it’s time to bring your business into the 21st century.
Onwards and upwards. No more Same Stuff Different Day garbage. Think differently, revolutionise your business with digital channels and opportunities for your business to flourish in 2016. The same activities from the last 10 years quite frankly aren’t going to cut it in 2016.
by Michael Cropper | Nov 6, 2015 | SEO |
What is the Yoast SEO Plugin
Before we get started, let’s first just look at what the Yoast SEO plugin actually is. This is the leading free plugin available in the WordPress space which is designed to allow you to customise the basic on-site technical SEO items on your website. This helps Google to crawl and index your website easier which in turn can help to increase visibility in the search engines. The Yoast SEO plugin powers over 22,000,000 websites to put its popularity into context. Quite simply, you would never use anything else, Yoast is awesome.
Installing the plugin is one thing. Knowing how to use the Yoast SEO plugin once installed is another. For many newbie webmasters, the Yoast SEO plugin can feel a little daunting at first yet it needn’t be when you know what you’re doing.
One important point to note about Yoast SEO though, and this is a big one, installing the Yoast SEO plugin on your WordPress website does not mean that you have taken care of your SEO. SEO is a little more complex than that… The Yoast SEO plugin simply means that your website isn’t technically bad and is not making life difficult for Google to crawl and understand the basic content on your website. If installing a single plugin on your website meant that you had “done SEO” then everyone would be doing it, right? Exactly. There is a lot more to SEO than just installing a plugin and making the options go green.
The Yoast SEO plugin is great, although it can mislead, not intentionally, newbies to SEO by thinking that if the page traffic light system is green then all is good and you can sleep easy at night. Green simply means that you have at least got the very basics in place. Red means that Google is not going to look too kindly on your website.
Ok, so now we’ve got that caveat out of the way, let’s take a look at how to get the most out of the Yoast SEO plugin and how you can work with this on a day to day basis. This type of work is not something we generally get involved with as it is simply too time consuming and hence costly for businesses. Instead, by us helping you help yourself, we can focus on the strategic side of your digital marketing.
General Settings Page
Your Information Tab
Website Name
The website name settings allow you to inform Google what your website is called. This is generally set to either your brand name as a business or if this is a personal blog, then your own personal name;

Company or Person
Self-explanatory, the company or person details allow you to specify this information to help Google understand who is behind the website. This information is often used to pull in various information from your website into the search results;
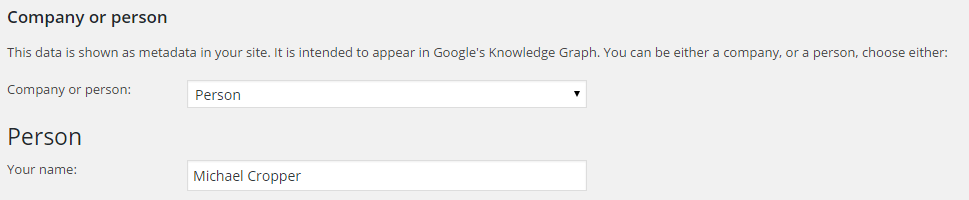
Webmaster Tools Tab
If you’re not using Google Webmaster Tools yet, what are you waiting for, get signed up at www.google.com/webmasters/tools/. This free tool will help you to understand how Google is viewing your business website. There are many ways for which you can verify your Google Webmaster Tools account, personally I prefer to use the Google Analytics option although if this is not possible for you then you can always use the meta tag verification option where you can place your code into this box here.
Security Tab
These settings allow authors and editors to redirect posts, noindex them and do other things which you might not want if you don’t trust your authors. Specifically these settings are really only relevant for websites with a large number of authors writing content on the website.
Titles & Metas Page
General Settings tab
You probably don’t need to change anything here so it would be recommended to leave these settings as they are out of the box unless you know what you are doing.
Homepage tab
These settings tell Google how your website should be displayed within their search results pages. It is important to fill this information out accurately so that Google understands what your website is about. It is important to note that you should keep your Meta Title set to around 65 characters and your Meta Description to around the 165 characters limit. Any more than this and your content will be snipped to fit within the standardised listings on Google. In the screenshot below, the Meta Title is the blue bit that you see on Google which is counted as a ranking signal and the Meta Description is the grey bit which is not counted as a ranking signal. This being said the Meta Description can allow you to entice potential customers to click through to your website when they see your listing alongside competitors.
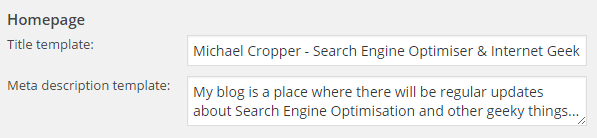

Post Types tab
Unless you are an advanced user and know what you are doing, then you should probably leave these settings as they are by default. Changing settings within here could result in your accidentally telling Google not to include your website in their search results which means that you will not receive any traffic from Google. Which is probably not something that you want to happen. For reference, here are what some of the settings mean;
- Noindex: Ticking this option will tell Google to not include these set of pages within their index. This can be used for certain Post Types which may include low quality content in the eyes of Google or duplicated content. There are valid reasons to use this, although in most cases this should be left unticked.
- Follow: This means that Google will follow the links that are included on this set of pages and pass authority to the pages that are being linked to. Generally speaking, there is no reason why you would ever nofollow any links on your own website.
- Show date in snippet preview: This option provides additional information to Google when they crawl your website which indicates to them when the blog post or page was published. This can be useful for certain Page Types such as blog posts although can be problematic for static content such as ecommerce products or service pages on your website.
Taxonomies tab
As above, it’s probably best you leave these settings as they come for all of the same reasons outlined above in the Post Types section. Taxonomies within WordPress are ways of categorising the content on your website.
Archives tab
As above, it’s probably best you leave these settings as they come. Most business websites do not need to do anything with these settings unless concerned about being penalised for duplicate content.
Other tab
There is very little that really needs customising here for the majority of WordPress websites. If you are thinking about customising any of these settings, it’s best you seek the advice of an expert. To understand some of the phrases listed within these settings, here is what they mean;
- Meta Keywords tag: This is a piece of code that is used within HTML which was originally designed to help to categorise pages by including keywords for what the page is about. Do not bother about this tab, Google and all major search engines completely ignore this information so you will be wasting your time filling this out.
Social Page
Accounts tab
For all of the social media channels you are active on, list your information in here. This helps Google to understand which social channels belong to your business.
Facebook tab
Open Graph tags help Facebook to generate key pieces of information about the page on your website that is being shared on Facebook which is then presented in an ideal way for your audience. For example, when you see an image and title nicely listed on Facebook when you have shared some content, this is due to these tags being used.
For websites with a high number of social shares then it is recommended to implement these settings as this will make any content that is shared on Facebook from your website look better than if this information wasn’t present.
Most of the settings are self-explanatory which helps, the one point which you may not have come across before relates to the Facebook Insights and Admins. Within your Facebook business page, you will have a specific App ID which can be used to input into this section which helps to gather data about content that is shared on Facebook throughout the Facebook network. For example, whenever someone shares content from your website onto Facebook, when this setting is configured then you can see the reach of your content within the Facebook Insights reports which can help to gather data about how your digital strategy is performing and most importantly which pages on your website are most popular on Facebook. This is a more advanced setting, so if you aren’t sure what you are doing here then it is best to seek the advice of an expert.
Twitter tab
The Twitter Cards tags do essentially the same thing as the Facebook Open Graph tags. They are designed to help Twitter understand what the content is about on the page that is being shared which can then be automatically used for generating the social media update and making this look good. Again, configure these settings accordingly if your website has a good amount of social shares.
Pinterest tab
This information is self-explanatory, configure these settings if you have a Pinterest account. Pinterest also uses the Open Graph tags which Facebook uses, so if you configure this information, make sure your setting within the Facebook tab are also configured correctly.
Google+ tab
This information again is self-explanatory, so configure the settings accordingly if your business is active on Google+, while it is still around.
XML Sitemaps page
An XML Sitemap is designed to help Google understand what content is on your website and easily identify all of the pages that they should be aware of. XML is a structured format which allows Google to understand specific information that relates to a specific page on your website. For example, some of this information includes the date the page was last changed which can help inform Google if they should re-crawl that specific page or not.
XML Sitemaps are essential for websites and it is important that your website has an automatically updating XML Sitemap so that whenever you make a change on your website then this is automatically reflected in your sitemap. Thankfully, WordPress handles this all automatically for you. For non-WordPress websites, there are free services such as Simple Sitemap Generator which allow you to quickly create a temporary XML sitemap while you work on building a more robust system and framework for your website to update all of this dynamically.
For the average business, you probably don’t need to configure any settings within here. Just make sure that you submit your XML Sitemap to Google via Google Webmaster Tools so that Google is aware of all of your content. There is a link provided to your sitemap for ease which is likely to sit at www.example.com/sitemap_index.xml
Advanced page
Breadcrumbs
The breadcrumbs settings within the Yoast SEO plugin is a little more advanced and requires a developer to set this up correctly. When this setting is turned on, and it is generally recommended that breadcrumbs are used as this helps massively with SEO, then you need to configure some PHP code and add a snippet to your WordPress theme. If this is a little beyond your level of expertise, then speak to an expert who can implement this for you.
Permalinks
Permalinks in WordPress are the URLs such as www.example.com/product-x/ for example. There are a lot of different types of permalinks throughout WordPress which are designed to show different content on your website.
RSS
Your RSS feed for your website can generally be found at www.example.com/feed which lists all of the recent blog posts on your website. This is useful for other systems to be able to pull in the content from your website onto theirs if needed.
For most users, customising the RSS feed is something that likely isn’t relevant as the default settings within Yoast SEO are more than sufficient.
Tools Page
There are a couple of handy tools within Yoast SEO, primarily the Bulk Editor and the File Editor. The Bulk Editor tool allows you to quickly change the meta titles and meta descriptions on your website in bulk without needing to go into each page to edit these individually.
The File Editor tool allows you to change important files such as your .htaccess file and robots.txt file from within the WordPress interface. These are for more advanced users, so if you aren’t sure what to do with these, then don’t use them and seek the advice of an expert.
Page SEO Settings
In addition to the default settings within Yoast SEO there are additional page and blog post specific settings which need checking over once you publish any page or blog post on your website. This information allows you to target specific keywords that are of interest for each of the individual pages on your website. It is important to note that these settings are the very basics to SEO, and simply turning these settings green does not mean that you now have a successful SEO strategy in place that is going to work for your business.
For the general settings tab on your new blog post or page, this allows you to focus on making sure the keywords you are targeting are within the key elements on the page. To do this, enter in the main keyword within your Focus Keyword settings tab then look to make sure that everything at least includes this target keyword as outlined on the page.
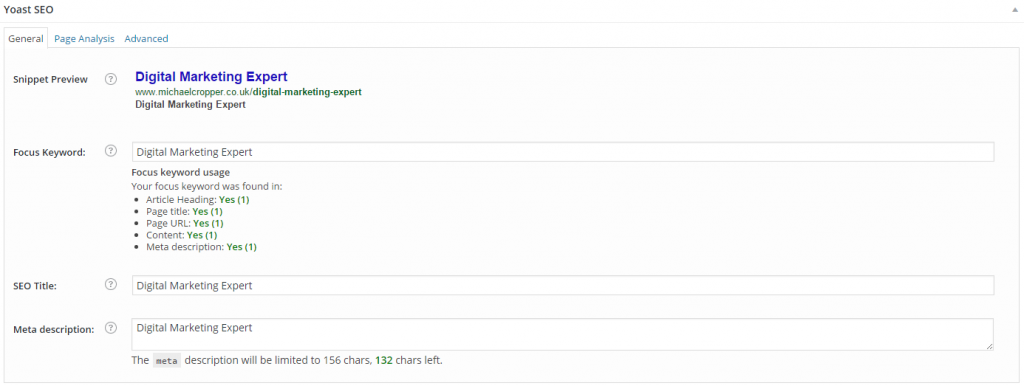
The next tab allows you to go into a bit more depth to make sure the content on the page is targeting this main keyword. The Page Analysis tab allows you to go into more detail to make sure the content you are producing is as good as it can be, with tips provided throughout;
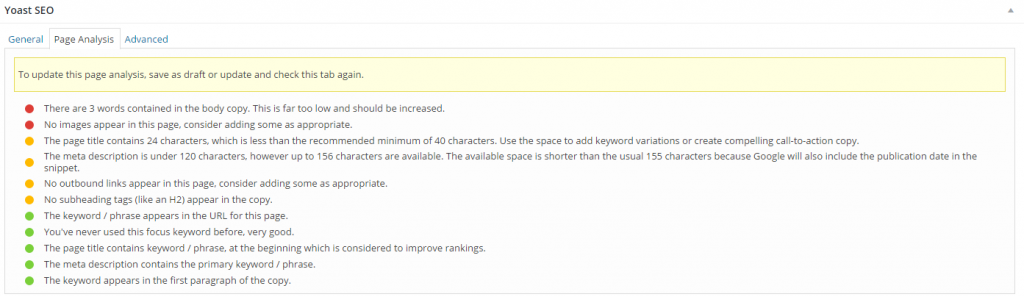
Again, it is important to note that this is simply a guide for what can be produced and should not be seen as a strict rule to follow. Use your intuition here to avoid overly focusing on SEO when you should be focusing on the user experience instead.
The Advanced settings tab on the page should not be touched unless you know what you are doing. These settings can quite easily tell Google to not crawl and index the new content that you have just creased which you probably don’t want to accidentally trigger.
Summary of Yoast SEO for WordPress
In summary, it is important to configure all of the settings within Yoast SEO which are relevant for your business. There are no one set of rules that apply to every single website as this can vary hugely from website to website. As such, review which settings are most appropriate to use for your own website and do not change any settings unless you are confident that you know what you are doing.
The settings and configuration options within Yoast SEO are the real basics for SEO. Implementing all of these basic settings is going to allow Google to at least understand the content on your website currently. The next step for SEO, and the work that is going to generate you some real results is focused around content marketing and promotion which is a much bigger topic to cover.
As always, if there are any questions specific to your business where you could use a bit of advice on the topic then get in touch and we’ll be happy to guide you through the process and show you where improvements can be made.